Building Blazingly Fast Websites Using The Speculation Rules API
The Speculation API is a browser feature that lets us define rules for speculative navigation. What this means is we are given a way that allows the browser to make educated guesses about what your user will do next and prepare the content in advance. For example; If the user is about to click a link, the browser can prefetch resources for that link. If the browser is confident enough, it might even prerender the entire page, making the navigation feel almost instant when the user does click the link.
By reducing the time it takes to load content, you can make your web apps feel significantly faster, which of course is always the defacto for a world class user experience.
Warning
The Speculation Rules API is only available in Chromium browsers (Chrome and Edge) while this means it may not benefit all your users, the Chromium engine does have a 74.15% global reach.
The Difference Between Prefetch and Prerender
#The Speculation API operates by defining speculation rules in your HTML. These rules tell the browser when and how to prefetch or prerender specific resources.
Prefetch
Downloads resources that may be needed soon for exampled a linked page and ensures the browser has assets ready in the cache when the user clicks the link they want to navigate to.
Prerender
This is a more resource intensive process. It fully renders a page in the background, so when a user navigates, they see the page instantly. It’s more resource-intensive but provides the smoothest experience.
Info
preload
, prefetch
, preconnect
are all attributes that are used with the <link rel="">
. For a while there was the option of prerender
but this has been superseded by The Speculation API which works totally independently of any of these attributes.
Browser Support And Detection
#To make sure you can see the benefits of speculative navigation in your browser, navigate to your browser settings to enable web page preloading. In Chrome, you can configure this by navigating to chrome://settings/performance/
and ensuring the Preload pages for faster navigation and searching setting is enabled.
You can progressively enhance a page to use speculation rules. Using this conditional logic, browsers that don’t support speculationrules
will ignore the rules and will skip prerendering or prefetching.
_10if (_10 HTMLScriptElement.supports &&_10 HTMLScriptElement.supports("speculationrules")_10) {_10// Speculation rules is supported_10}
Prefetch HTML Setup
First, we define speculation rules in our HTML to tell the browser to prefetch resources for specific links:
_18<!DOCTYPE html>_18<html lang="en">_18 <head>_18 <title>Dog Adoption</title>_18 <script type="speculationrules">_18 {_18 "prefetch": [_18 { "source": "list", "urls": ["/dog/1", "/dog/2", "/dog/3"] }_18 ]_18 }_18 </script>_18 </head>_18 <body>_18 <a href="/dog/1">View Dog 1</a>_18 <a href="/dog/2">View Dog 2</a>_18 <a href="/dog/3">View Dog 3</a>_18 </body>_18</html>
In this example the "prefetch"
rule tells the browser to fetch the resources for /dog/1
, /dog/2
, and /dog/3
in advance. This means that when the user clicks on one of the links, the browser already has the necessary data cached.
Integrating with React
This is also possible when using frontend frameworks such as React. Combining React Router with the Speculation API would look something like this.
_28import React from "react";_28import { BrowserRouter, Routes, Route, Link } from "react-router-dom";_28_28const Home = () => (_28 <div>_28 <h1>Welcome to the Dog Adoption Site!</h1>_28 <ul>_28 <li><Link to="/dog/1">View Dog 1</Link></li>_28 <li><Link to="/dog/2">View Dog 2</Link></li>_28 <li><Link to="/dog/3">View Dog 3</Link></li>_28 </ul>_28 </div>_28);_28_28const DogProfile = ({ id }) => {_28 return <h2>Details for Dog {id}</h2>;_28};_28_28const App = () => (_28 <BrowserRouter>_28 <Routes>_28 <Route path="/" element={<Home />} />_28 <Route path="/dog/:id" element={<DogProfile />} />_28 </Routes>_28 </BrowserRouter>_28);_28_28export default App;
To implement the Speculation API, we simply add a <script>
tag to our index.html
file with speculation rules.
_10<script type="speculationrules">_10{_10 "prefetch": [_10 { "source": "list", "urls": ["/dog/1", "/dog/2", "/dog/3"] }_10 ]_10}_10</script>
Using Prerendering
Prefetching is great for small assets, but for maximum speed, prerendering is the real MVP. You can specify rules to prerender a page like so:
_10_10<script type="speculationrules">_10{_10 "prerender": [_10 { "source": "list", "urls": ["/dog/1"] }_10 ]_10}_10</script>
Of course we can also achieve this with Javascript by doing the following.
_12const scriptEl = document.createElement("script");_12scriptEl.type = "speculationrules";_12const rules = {_12 prerender: [_12 {_12 "source": "list",_12 "urls": ["/dog/1"] _12 },_12 ],_12};_12scriptEl.textContent = JSON.stringify(rules);_12document.body.append(scriptEl);
When a user visits the homepage, the browser can fully render the /dog/1
page in the background. If they click on that link, the page is already rendered and loads instantly.
Tip
Prerendering uses more browser resources, so use it selectively for high-priority pages or links you’re confident users will visit.
Prerendering Specific Links Only
#It can be wasteful to prerender all links on our site, it makes more sense that we target the links that know take longer to render than others or more commonly clicked links. This is where the property href_matches
comes in to specify which pages to prerender unlocking a powerful way to specify which pages to prerender.
The where
property is used to specify conditions for prerendering. The and
property is used to combine multiple conditions.
_14<script type="speculationrules">_14 {_14 "prerender": [_14 {_14 "where": {_14 "and": [_14 { "href_matches": "/adopt/dog/1" },_14 { "not": { "href_matches": "/logout" } }_14 ]_14 }_14 }_14 ]_14 }_14</script>
Prerendering CSS Selectors
#Using the selector_matches
property allows us to specify which pages to prerender based on CSS selectors. Depending on your use case, this can lead to declarative code like this:
_18<script type="speculationrules">_18 {_18 "prerender": [_18 {_18 "where": {_18 "and": [_18 { "href_matches": "/*" },_18 {_18 "not": {_18 "selector_matches": ".prerender-link"_18 }_18 }_18 ]_18 }_18 }_18 ]_18 }_18</script>
Prerendering Eagerness
#The speculation rules API allows you to specify an eagerness
setting to control when a page is prerendered.
immediate
The page is prerendered or prefetched immediately.
moderate
The page is prerendered or prefetched when the user hovers over a link for 200 milliseconds.
conservative
The page is prerendered or prefetched when the user initiates a click on the link.
Info
You might be wondering “What’s the point of the conservative
setting?” since clicking on a link will open the following page anyway.
In general a click or touch event is fired on the “release” part of the interaction. The key here is that with the speculation rule the next page will start loading in the background as soon as the mouse button goes down or as soon as the tap interaction starts on a mobile device.
In general a click or touch event is fired on the “release” part of the interaction. The key here is that with the speculation rule the next page will start loading in the background as soon as the mouse button goes down or as soon as the tap interaction starts on a mobile device.
_12<script type="speculationrules">_12 {_12 "prerender": [_12 {_12 "where": {_12 "href_matches": "/*"_12 },_12 "eagerness": "moderate"_12 }_12 ]_12 }_12</script>
Tips For Success
#While the Speculation API is powerful, there are some best practices and pitfalls to keep in mind:
Do’s
Prefetch links that users are likely to click but don’t require high resource consumption.
For prerendering, focus on the pages most critical to your app’s flow.
Use tools like Chrome DevTools or Lighthouse to analyse the effectiveness of your prefetching and prerendering strategies.
Don’ts
Don’t prefetch every link. It can overwhelm the browser and slow down the user’s current experience.
Be careful with prerendering pages with heavy content. It can degrade performance on lower-end devices.
Speculation rules might inadvertently leak sensitive data about user behaviour to analytics tools, so beware to review your rules carefully.
Conclusion
#The Speculation API is a powerful tool for improving user experience by making your web app faster and more responsive. By letting the browser prefetch and prerender resources based on user behaviour, you can significantly cut down on load times and delight your users.
We’ve seen how to set up speculation rules in HTML and integrate them with a React app. Now, it’s your turn to experiment and see how the Speculation API can improve your own projects. As browsers continue to adopt this feature, it’s worth staying ahead of the curve and integrating it into your performance optimisation strategies.
Halpy coding!
Reinforce Your Learning
One of the best ways to reinforce what your learning is to test yourself to solidify the knowlege in your memory.
Complete this 3 question quiz to see how much you remember.
Performance
Thanks alot for your feedback!
The insights you share really help me with improving the quality of the content here.
If there's anything you would like to add, please send a message to:
[email protected]Was this article this helpful?
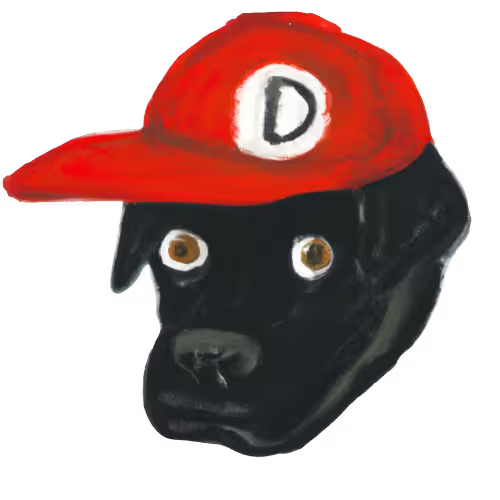
About the author
Danny Engineering
A software engineer with a strong belief in human-centric design and driven by a deep empathy for users. Combining the latest technology with human values to build a better, more connected world.