Utilising ARIA Attributes For Accessible React Components
To avoid these issues, developers should understand when and how to apply ARIA attributes effectively. This guide provides a comprehensive checklist to help you correctly implement ARIA in your React components. We’ll explore best practices, common pitfalls, and practical examples to ensure your components are fully accessible.
Using ARIA the Right Way in React
#ARIA is a powerful tool, but it should always be used as a last resort. Whenever possible, prefer native HTML elements that already have built-in accessibility support. HTML provides meaningful roles and behaviours automatically, reducing the need for additional ARIA attributes. For example, instead of using a <div>
with role="button"
, simply use a <button>
, which is already keyboard-navigable and screen reader-friendly.
Another key principle of ARIA is keeping attributes in sync with component state. If an interactive component changes state, the corresponding ARIA attributes such as aria-expanded
or aria-hidden
should be updated dynamically. Failing to do so can lead to incorrect information being conveyed to screen readers, creating a frustrating user experience.
Finally, test your ARIA implementations using screen readers and accessibility tools. While adding ARIA attributes might seem straightforward, the only way to truly verify their effectiveness is to test how they behave in real-world scenarios. Tools like VoiceOver (Mac), NVDA (Windows), ChromeVox, and axe DevTools can help identify potential issues.
Ensure Proper Labelling with aria-label
and aria-labelledby
#ARIA labelling is essential for elements that lack visible text. Screen readers need descriptive labels to communicate the purpose of an element. If an interactive element, such as an icon button, does not have visible text, you should provide an accessible name using aria-label
.
For example, if you have a button that closes a modal but only contains an SVG icon with no visible text, it won’t be accessible by default. Adding aria-label="Close modal"
ensures that screen readers can correctly interpret the button’s function:
_10<button aria-label="Close modal" onClick={handleClose}>_10 <svg> {/* Close icon */} </svg>_10</button>
If the button is associated with a visible text element, use aria-labelledby
instead. This links the element to an existing label, avoiding redundancy and improving clarity.
_10<h2 id="modal-title">Delete Confirmation</h2>_10<button aria-labelledby="modal-title">Close</button>
This approach makes sure that assistive technologies associate the button with the heading, providing context without requiring additional labels.
Tip
Avoid using both aria-label
and aria-labelledby
on the same element, as they can conflict and confuse screen readers.
Hide Non-Essential Content with aria-hidden
#Some UI elements, such as decorative icons or background images, should not be announced to screen readers. The aria-hidden="true"
attribute ensures that assistive technologies ignore these elements.
For example, if an SVG icon is purely decorative and does not add meaning, it should be hidden from screen readers:
_10<svg aria-hidden="true"> {/* Decorative icon */} </svg>
However, aria-hidden="true"
must not be used on interactive elements such as buttons or links. If you apply aria-hidden="true"
to a button, it becomes completely invisible to screen readers, making it impossible for users with disabilities to interact with it.
Tip
Never use aria-hidden="true"
on elements that users need to interact with, such as buttons, links, or form fields.
Use the Correct role
Attribute for Interactive Components
#HTML provides built-in semantic roles for elements like <button>
, <input>
, and <nav>
, but some complex components need additional clarification using ARIA role
attributes.
For example, modals should be announced as dialogs so that assistive technologies recognise them as separate from the main page content. Adding role="dialog"
and aria-modal="true"
makes this explicit:
_10<div role="dialog" aria-modal="true" aria-labelledby="modal-title">_10 <h2 id="modal-title">Subscribe to our newsletter</h2><button onClick={handleClose}>Close</button>_10</div>
Using aria-labelledby="modal-title"
links the modal to its heading, ensuring screen readers announce the purpose of the dialog when it appears.
Tip
Avoid using roles unnecessarily. For example, adding role="button"
to a <button>
is redundant because <button>
already has this role by default.
Indicate Component State with aria-expanded
#Interactive elements that expand or collapse content, such as dropdown menus, accordions, and disclosure widgets, should communicate their state to screen readers using aria-expanded
.
For example, when a dropdown menu is toggled open, aria-expanded
should reflect its state dynamically:
_11<button_11 aria-expanded={isOpen}_11 aria-controls="dropdown-menu"_11 onClick={() => setIsOpen(!isOpen)}_11>_11 Menu_11</button>_11<ul id="dropdown-menu" hidden={!isOpen}>_11 <li><a href="/profile">Profile</a></li>_11 <li><a href="/settings">Settings</a></li>_11</ul>
The aria-controls="dropdown-menu"
attribute links the button to the menu, while aria-expanded={isOpen}
ensures assistive technologies understand whether the menu is open or closed.
Tip
Forgetting to update aria-expanded
dynamically when the menu state changes. If the attribute remains false
while the menu is visible, screen readers will announce incorrect information.
Announce Dynamic Content Updates with aria-live
#When content updates dynamically screen readers may not detect these changes unless aria-live
is used.
For example, in a form submission process, using aria-live="polite"
ensures that success or error messages are announced automatically without disrupting the user’s workflow:
_10<p aria-live="polite">{isSubmitting ? "Submitting form..." : "Form submitted successfully!"}</p>
If the update is urgent such as an error message that needs immediate attention, aria-live="assertive"
forces an immediate announcement:
_10<p aria-live="assertive">Error: Please enter a valid email address.</p>
Tip
Using aria-live="assertive"
unnecessarily. This can be intrusive and disruptive, interrupting the user’s current task.
Conclusion
#ARIA is a powerful but complex tool for improving accessibility in React applications. When used correctly, it enhances custom UI components, making them usable for a broader audience. However, misusing ARIA can create unnecessary barriers.
- Use semantic HTML whenever possible, applying ARIA only when necessary.
- Ensure all interactive elements are keyboard-accessible—test using
Tab
andEnter
. - Dynamically update ARIA attributes
aria-expanded
,aria-hidden
, etc. when component state changes. - Test ARIA attributes with screen readers like VoiceOver (Mac), NVDA (Windows), and ChromeVox.
- Avoid redundant or incorrect ARIA roles that duplicate built-in HTML functionality.
- By integrating these best practices, you’ll ensure that your React applications are more accessible, user-friendly, and inclusive for everyone.
Halpy coding,
Quiz Time!
#Reinforce Your Learning
One of the best ways to reinforce what your learning is to test yourself to solidify the knowlege in your memory.
Complete this 8 question quiz to see how much you remember.
React
Thanks alot for your feedback!
The insights you share really help me with improving the quality of the content here.
If there's anything you would like to add, please send a message to:
[email protected]Was this article this helpful?
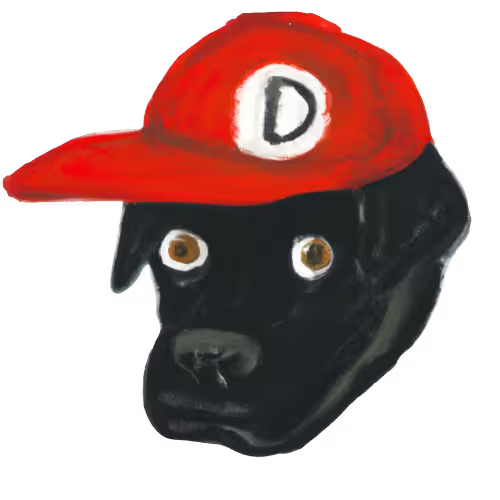
About the author
Danny Engineering
A software engineer with a strong belief in human-centric design and driven by a deep empathy for users. Combining the latest technology with human values to build a better, more connected world.