Conditionally Spreading Objects With JavaScript
In JavaScript, if you want to populate an object with some properties from another object, you can use the spread operator ...
to do so.
_11_const_ obj = {_11 a: 1,_11 b: 2,_11 c: 3_11};_11_11_const_ newObj = {_11 ...obj_11};_11_11console.log(newObj);// { a: 1, b: 2, c: 3 }
But what if you want to conditionally spread the properties of an object? For example, you want to spread the properties of an object only if a certain condition is met.
Short-circuiting the spread operator
#To shorten the spread operator, we can use the &&
operator. If the condition is met, the object properties will be spread. Otherwise, it will be skipped.
Look at this example:
_10const isActive = true;_10const user = { name: "Amit", age: 30};_10const activeUsers = { ...isActive && user};_10_10console.log(activeUsers);// { name: 'Amit', age: 30 }
In the example above, the user
object only receives "spread" if isActive
is true
. The logical &&
evaluates the operands from left to right, and returns immediately with the value of the first falsy operand it encounters. If all values are truthy, the value of the last operand is returned.
But beware! The first operand must be a boolean value. So, if you want to use a variable as the first operand, you have to convert it to a boolean value using, let’s say, the Boolean()
function.
_10_10const user = { name: "Amit", age: 30};_10const isActive = Boolean(user.name); _10const activeUsers = { ...isActive && user};_10_10console.log(activeUsers);// { name: 'Amit', age: 30 }
Javascript
Thanks alot for your feedback!
The insights you share really help me with improving the quality of the content here.
If there's anything you would like to add, please send a message to:
[email protected]Was this article this helpful?
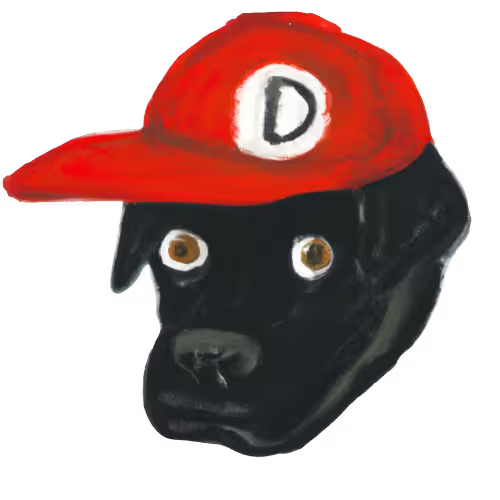
About the author
Danny Engineering
A software engineer with a strong belief in human-centric design and driven by a deep empathy for users. Combining the latest technology with human values to build a better, more connected world.