What is Metaprogramming in JavaScript?
If you’ve ever wondered how frameworks like React can dynamically manipulate components, or how TypeScript enhances JavaScript's capabilities, then understanding metaprogramming is a fantastic next step in your frontend development journey.
In this article, we’ll explore what metaprogramming is, why it’s useful, and how to harness its power using the Reflect and Proxy objects introduced in ES6 (ECMAScript 2015). By the end, you’ll have a solid understanding of how these concepts work and hopefully a spark which will carry over to some creativity in your own coding practices.
What is Metaprogramming?
#Metaprogramming might sound like something out of a sci-fi fantasy novel, but it's very much a real and practical programming technique. In the simplest terms, metaprogramming is the practice of writing code that can manipulate other code. Imagine writing a program that can read, modify, analyse, or even generate other programs. It’s like a magician pulling a rabbit out of a hat, but instead, you’re pulling out functions.
You can use it to automatically produce code structures or routines and in turn modify existing objects, methods, or classes during the execution of the program.
This level of abstraction allows developers to create more flexible and dynamic applications.
With the introduction to ES6, JavaScript introduced the Reflect and Proxy objects, which make metaprogramming significantly more accessible and powerful. While the overarching concept of metaprogramming wasn't introduced with ES6, the new APIs have greatly simplified its implementation.
Key Concepts in Metaprogramming
Reflection
Reflection is a powerful concept that allows a program to inspect and modify its own structure and behaviour at runtime Imagine being able to look at your class from the inside out and tweak its properties or methods as you see fit. It’s like giving your code a mirror to look at itself and decide what to do based on what it sees.
There are three main sub-branches to reflection which are:
Introspection: This allows access to the internal properties of a program, such as variables, methods, and classes, which can then be analysed or used dynamically.
Intercession: This involves acting on behalf of another piece of code, often by intercepting operations or wrapping functionality to modify its behaviour without altering the original code by way of wrapping, trapping, and intercepting.
Self-Modification: This is when code modifies itself during execution. Although powerful, this is a fairly advanced and complicated technique which can be mitigated in favour of safer, more predictable methods.
For instance, in JavaScript, you can loop over the properties of an object using Object.keys()
or check if a certain method exists using typeof object.method === 'function'
.
Beyond inspection, reflection also allows you to modify the structure of objects or classes. This means you can dynamically add, remove, or alter methods and properties.
Imagine you're building a form handler that needs to process different types of inputs dynamically. With reflection, you could write a single function that adjusts its behaviour based on the type and properties of the input fields it’s dealing with, rather than writing separate handlers for each case.
Proxies
These are like middlemen between your code and the objects it interacts with. They allow you to intercept and redefine operations performed on objects, such as property access, assignment, or deletion.
A Proxy wraps around an object and lets you intercept operations on that object. For instance, you can control what happens when a property is accessed or modified.
By intercepting these operations, you can add custom behaviour. For example, you might enforce validation rules when properties are set, or log every time a certain property is accessed.
A real world example would be where you need to validate user input across various forms in your application. By using a Proxy, you can enforce validation rules directly at the point where data is assigned to form fields, ensuring that invalid data never makes it past the assignment stage.
This concept allows you to create a wrapper for an object, enabling you to intercept and redefine fundamental operations like property lookup, assignment, and enumeration.
Decorators
This is more of a design pattern that allows you to add new behaviour or functionality to functions, methods, or classes without modifying their structure. They act like wrappers, enhancing the original functionality while keeping the core logic intact.
Decorators allow you to "decorate" a function or a method with additional features. For instance, you might want to log when a function is called, measure its execution time, or enforce access control.
Despite adding new behaviour, decorators do not alter the original structure of the function or method. This is particularly useful for keeping your code modular and clean.
In a React application, you might use decorators to automatically bind methods to their class instances, or to enforce certain security checks before a method can execute. This can be particularly useful in large applications where consistency and security are key concerns.
This design pattern lets you attach additional behavior to an object without modifying its structure. Think of it like wrapping a gift; you still retain the original item, but it’s dressed up for a special occasion!
Metaprogramming with Proxies
#Let’s dive into a practical example of using Proxies for metaprogramming in JavaScript. Imagine we’re building a simple data validation system for a form input. We want to ensure that all inputs are strings, and we’ll use a proxy to enforce this.
Step 1: Create a Target Object
First, let’s create an object that will serve as our target.
_10const target = {_10 name: '',_10 email: ''_10};
Step 2: Create a Proxy
Now let’s create a proxy. This will allow us to intercept operations on our target object.
_11const handler = {_11 set(target, property, value) {_11 if (typeof value !== 'string') {_11 throw new TypeError(`${property} must be a string`);_11 }_11 target[property] = value;_11 return true;_11 }_11};_11_11const proxy = new Proxy(target, handler);
Step 3: Test the Proxy
Now, let’s try setting some properties on our proxy:
_10try {_10 proxy.name = "John Doe"; // Works fine!_10 proxy.email = 12345; // Throws a TypeError: email must be a string_10} catch (e) {_10 console.error(e);_10}
With this setup, any attempt to set a property to a non-string value will throw an error, ensuring our inputs are validated before they even reach the rest of the application.
So where does metaprogramming come into play in real-world applications? Let’s look at a scenario using React, our beloved library.
Dynamic Component Generation
In a React application, we might dynamically generate components based on user roles. With metaprogramming, we can use Proxies to streamline this process. Suppose we have a set of permissions and associated components:
_14const permissions = {_14 admin: <AdminComponent />,_14 user: <UserComponent />,_14 guest: <GuestComponent />_14};_14_14const proxyPermissions = new Proxy(permissions, {_14 get(target, prop) {_14 if (!target[prop]) {_14 return <NotFoundComponent />;_14 }_14 return target[prop];_14 }_14});
Here, if a user has an unrecognized permission, we dynamically return a "Not Found" component. This keeps our code clean and minimizes the need for repetitive conditional statements.
Practical Tips and Best Practices
#- Keep it Simple: Metaprogramming can get complex quickly, so always prioritize readable and maintainable code. Don’t over-engineer solutions, especially when simpler methods can achieve the same outcome.
- Understand the Trade-offs: While metaprogramming can increase flexibility, it can also make debugging more challenging. Ensure that you weigh the benefits against the potential complexities it adds.
- User-Centric Development: Always keep user needs in mind. The goal of using metaprogramming should be to enhance user experience and streamline functionalities that provide real value.
Metaprogramming isn’t just a buzzword; it’s a powerful paradigm that, when used correctly, can greatly enhance the flexibility and dynamism of your JavaScript applications. By mastering concepts like proxies and reflection, you’re setting yourself up for success in creating complex, user-friendly applications, particularly in the rich ecosystem of React and TypeScript.
Hopefully, this exploration piqued your interest and gave you practical insights into applying metaprogramming in your projects. If you're eager to learn more about JavaScript and frontend engineering, stick around for our next posts. Until then, keep coding and experimenting! Happy coding!
Reinforce Your Learning
One of the best ways to reinforce what your learning is to test yourself to solidify the knowlege in your memory.
Complete this 6 question quiz to see how much you remember.
Javascript
Thanks alot for your feedback!
The insights you share really help me with improving the quality of the content here.
If there's anything you would like to add, please send a message to:
[email protected]Was this article this helpful?
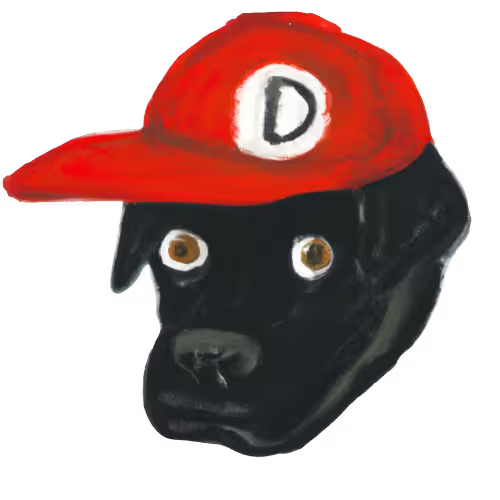
About the author
Danny Engineering
A software engineer with a strong belief in human-centric design and driven by a deep empathy for users. Combining the latest technology with human values to build a better, more connected world.