Typescript: An Introduction
In the 2022 Stack Overflow survey, Typescript placed top 5 in the list of languages most loved by engineers and 2nd in the list for what engineers want to be using.
TypeScript is a strongly-typed superset of JavaScript that provides type safety, which means that it helps you to catch errors at compile time, rather than runtime. This makes it easier to write and maintain by improving code readability and maintainability. It does this by adding type annotations to your code which means you make it easier for other engineers to understand what types of values, functions and variables are expecting and returning.
What’s TypeScript?
#TypeScript was originally developed by Microsoft back in 2012. Syntactically it’s very similar to Javascript. In fact, all JavaScript is valid as TypeScript code. The TypeScript compiler simply compiles TypeScript code into JavaScript which is interpreted by the browser as plain ol’ vanilla JavaScript. Essentially TypeScript is just JavaScript, with static typing.
What’s Static Typing?
#A language is statically typed if the type of a variable is known at compile time, before the code is executed.
JavaScript is dynamically typed the interpreter assigns variables a type at runtime based on the variable's value after the code is executed.
Info
Compile-time and runtime are two programming terms. Compile-time is when the source code turns into executable code. Runtime is when the executable code starts running.
Let’s look at an example to understand what this really means.
_10let value = 5;_10value = "hello";
Here, the type of value
changes from a number
to a string
. In most circumstances this is probably not a wise idea and is likely to cause an error in our code at a later point, for example:
_10let value = "5";_10console.log(value + 2);
We would probably want the output to be “7” of this operation, right? In the wacky world JavaScript, this won’t be the case. Instead the output is “52”.
We are adding is a number
to a string
so the output will be converted to a string. JavaScript politely concatenates the two strings together, and the result is “52”.
In TypeScript, this is type of operation is forbidden. Our IDE will show us an error message while we write the code, or it will throw an error when we go to compile our TypeScript code to JavaScript.
_10let value:number = 5;_10value = "hello"; // error: Type '"hello"' is not assignable to type 'number'
If you use JavaScript, you’re not likely to know what the type for some object is. It’s possible you won’t realise what methods a certain object has, or even what fields it has. It will run just fine, until you need to use the result of that property, causing a semantic error.
Info
Semantic errors and logical errors are the same. Your code is correct and adheres to the correct syntax, but doesn't do what you think it does.
_15class MyClass {_15_15 private value: number;_15_15 constructor(value: number) {_15 this.value = value;_15 }_15_15 getNumber(): number {_15 return this.value;_15 }_15}_15_15let object = new MyClass(5);_15console.log(object.number); //"undefined"
With TypeScript, the compiler tells you what the problem is, so you are immediately aware that the code you’re writing doesn’t work. A few seconds of specifying types will save you from an hour of debugging.
_15class MyClass {_15_15 private value: number;_15_15 constructor(value: number) {_15 this.value = value;_15 }_15_15 getNumber(): number {_15 return this.value;_15 }_15}_15_15let object = new MyClass(5);_15console.log(object.number); // compiler error: Property 'number' does not exist on type 'ExampleCla
Enums
#Enums allow a developer to define a set of named constants. Using enums can make it easier to document intent, or create a set of distinct cases. TypeScript provides both numeric and string-based enums. TypeScript uses syntactical sugar to make it work much better.
_10_10enum Colour {_10 Red,_10 Green,_10 Blue,_10}_10_10let c: Colour = Colour.Green;_10c = "Orange" // "Orange isn't in the Colours enum, so TypeScript will be upset.
You can do something similar in Javascript, but it doesn’t really work.
_10_10_10var Color = {_10 Red: 0,_10 Green: 1,_10 Blue: 2,_10}_10_10var c = Color.Green;_10c = 6839; // You can do this, but it will cause problems in the logic of our code "
Literal Types
#TypeScript supports string
, boolean
and number
literal types. A literal type defines a type that accepts specified string literal. Use the string literal types with union types and type aliases to define types that accept a finite set of string literals.
_10type Animal = "Dog" | "Monkey"_10_10const animal: Animal = "Dog" // yay!_10const anotherAnimal: Animal = "Swan" // compiler error, an animal can only be a "Dog" oir "Monkey"
Interfaces
#You can enforce the structure of certain elements using interfaces. An interface defines the syntax that any entity must adhere to, you can think of it as a kind of contract.
_16type Specie = "Dog" | "Monkey"_16interface Animal {_16 name: string;_16 says: string,_16 specie: Specie,_16 talk: () => void_16 _16}_16_16const animal: Animal = {_16 type: "Dog", // could also be Specie.Dog_16 name: "Bruce",_16 says: "woof"_16}_16_16console.log(`${animal.name} the ${animal.specie} says "${animal.says}"!`); // Bruce the Dog says "woof"!
Typescript is a really great contribution to the JavaScript ecosystem. It offers many features the biggest one we detail is static typing which allows you and your team to work quicker and prevent regressions by being warning you about potential bug causing code via IDE auto-completion, type checking, and code navigation. It’s backwards compatible meaning it’s easy for to adopt without having to rewrite your entire codebase and supports object-oriented programming features such as classes, interfaces, and inheritance, making it easier for your team to write clean, maintainable code.
At the time of writing, TypeScript isn’t supported by Node.js, neither is it supported by any Internet browsers, so to use it, you’ll need to compile it to JavaScript which your can do easily with tsc. Newer runtimes such as Deno and Bun do support it out of the box, meaning TypeScript isn’t going anywhere, so try it out and start making your life a little easier!
Javascript
Thanks alot for your feedback!
The insights you share really help me with improving the quality of the content here.
If there's anything you would like to add, please send a message to:
[email protected]Was this article this helpful?
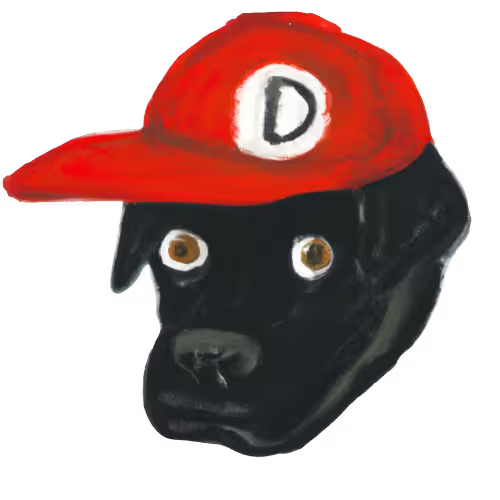
About the author
Danny Engineering
A software engineer with a strong belief in human-centric design and driven by a deep empathy for users. Combining the latest technology with human values to build a better, more connected world.